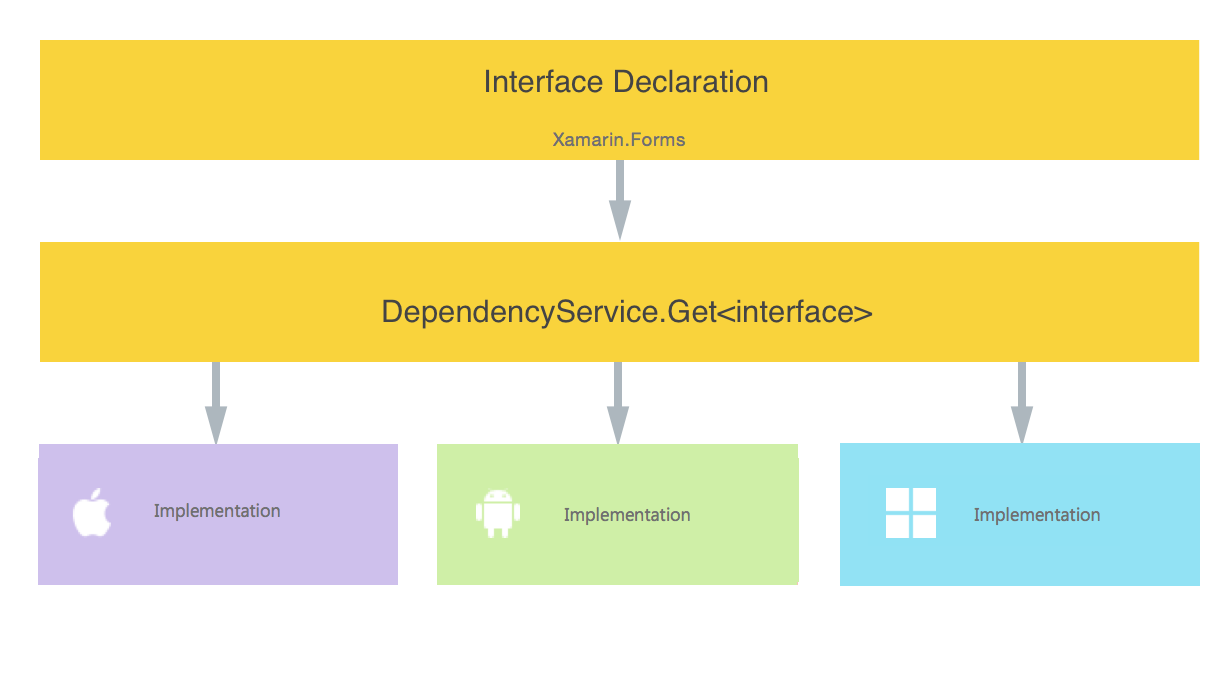
When working in Xamarin cross-platform, we sometimes want to show different behaviors on different platforms. For this, Xamarin offers us a solution with dependency services. It is quite simple to use. I made an example for this. Of course, you can follow these examples by following the lessons of Yiğit Özaksüt’s Xamarin Trainings. To watch Xamarin the Microsoft Open Academy course, you can follow the link to YouTube or Xamarin Turkey website.
I’ll tell you that in this example we run cross-platform, so you need to add some code to ensure that the same code works everywhere, as well as for platforms. To do this, we first need to create an interface called “helper” in the portable layer of our project. With this interface, we have to define this interface as we want to do the operations specifically for the platforms. In the example we will do now, if our project is working in an android environment, we will make an application that returns “1” to the screen if “0” is working on iOS.
First we need to open an interface to help us in the portable layer. We will later implement this interface as inheritance on other platforms. I will give this interface file the name “Helper” to help us. If we want to do what we want to do later, we need to define a function accordingly. Since I’m going to get a number from the platforms, I’m creating a function accordingly.
public interface IDeviceHelper { int GetDeviceNumber(); }
After creating this interface, I create another class called “Helper” on every platform to get numbers from other platforms. We implement these classes in such a way as to inherit the interfacial inheritance we create in the portable part. I will return 0 on Android.
[assembly:Xamarin.Forms.Dependency(typeof(DeviceHelper))] namespace XamarinDependency.Droid.Helper { public class DeviceHelper : IDeviceHelper { public int GetDeviceName() { return 0; } } }
Of course, we need to write some code at the beginning of the page to indicate that this class is a dependency services. We absolutely must do this. We need to do the same thing for the iOS platform after we have 0 on the Android platform. Of course we need to specify that there is a dependency services.
[assembly:Xamarin.Forms.Dependency(typeof(DeviceHelper))] namespace XamarinDependency.iOS.Helper { public class DeviceHelper : IDeviceHelper { public int GetDeviceNumber() { return 1; } } }
After we have done this, we need to write some code in the Main Page in the App class in the portable part. We are defining a variable named number to be the number. We will get this variant from a dependency services class, so we use the get method and give whatever type of interface we get the type of this method. Then we choose what we want from the functions on the interface.
namespace XamarinDependency { public class App : Application { public App() { int number = DependencyService.Get<IDeviceHelper>().GetDeviceNumber(); MainPage = new Sayfa(number); } } }
Subsequent classical Xamarin operations. If you want to access the project via github, you can access from here. If you have any questions, you can post or comment.
Leave a Reply