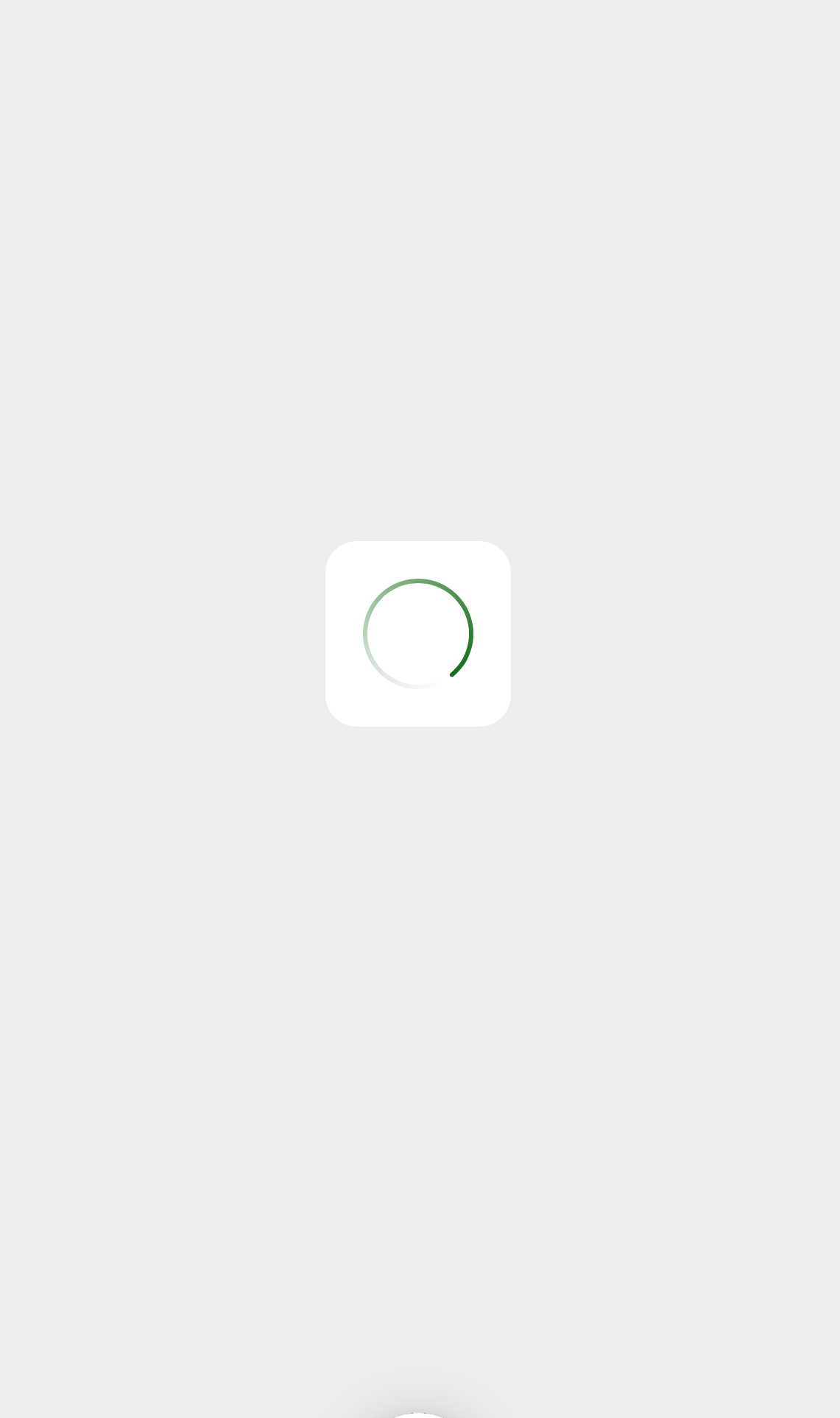
Hello friends, in this article, we will talk about how we can create a common waiting screen with Swift in our iOS projects. Actually, there are many ways to do this. These;
- Using a pod
- Writing extension to UIView
- Writing a class
Using Pod
Using a pod for the loading screen is one of the simplest and easiest ways. The pod we use the most for this job is SVProgressHud. You can view it here. You can simply load loading on your screen. Its output is as follows.
Writing Extension to UIView
This is one of the most logical ways if you want to put a separate view yourself here. Here, when you call the showLoading function in the View at the top of your ViewController, you will see a Loading icon with a blurred backside.
// // UIView+Extension.swift // OSMovie // // Created by Omer Sezer on 8.03.2021. // import UIKit extension UIView { func showLoading() { let blurLoader = BlurLoader(frame: frame) self.addSubview(blurLoader) } func hideLoading() { if let blurLoader = subviews.first(where: { $0 is BlurLoader }) { blurLoader.removeFromSuperview() } } } class BlurLoader: UIView { var blurEffectView: UIVisualEffectView? override init(frame: CGRect) { let blurEffect = UIBlurEffect(style: .dark) let blurEffectView = UIVisualEffectView(effect: blurEffect) blurEffectView.frame = frame blurEffectView.autoresizingMask = [.flexibleWidth, .flexibleHeight] self.blurEffectView = blurEffectView super.init(frame: frame) addSubview(blurEffectView) addLoader() } required init?(coder aDecoder: NSCoder) { fatalError("init(coder:) has not been implemented") } private func addLoader() { guard let blurEffectView = blurEffectView else { return } let activityIndicator = UIActivityIndicatorView() if #available(iOS 13.0, *) { activityIndicator.style = .large } else { activityIndicator.style = .gray } activityIndicator.frame = CGRect(x: 0, y: 0, width: 50, height: 50) blurEffectView.contentView.addSubview(activityIndicator) activityIndicator.center = blurEffectView.contentView.center activityIndicator.startAnimating() } }
The screen output is as follows.
Writing Class
Another method we use is to create a class, define it with a static variable and display it at the top layer of the screen. Just write the code below for this.
public class LoadingOverlay{ var overlayView = UIView() var activityIndicator = UIActivityIndicatorView() class var shared: LoadingOverlay { struct Static { static let instance: LoadingOverlay = LoadingOverlay() } return Static.instance } public func showOverlay() { if let appDelegate = UIApplication.shared.delegate as? AppDelegate, let window = appDelegate.window { overlayView.frame = CGRect(x: 0, y: 0, width: 80, height: 80) overlayView.center = CGPoint(x: window.frame.width / 2.0, y: window.frame.height / 2.0) overlayView.backgroundColor = .blue overlayView.clipsToBounds = true overlayView.layer.cornerRadius = 10 activityIndicator.frame = CGRect(x: 0, y: 0, width: 40, height: 40) activityIndicator.style = .whiteLarge activityIndicator.center = CGPoint(x: overlayView.bounds.width / 2, y: overlayView.bounds.height / 2) overlayView.addSubview(activityIndicator) window.addSubview(overlayView) activityIndicator.startAnimating() } } public func hideOverlayView() { activityIndicator.stopAnimating() overlayView.removeFromSuperview() } }
After that, just run the following line of code where you want to use it.
LoadingOverlay.shared.showOverlay()
You can reach the project here. If you have questions, you can ask by mail or comment. Good work.
Leave a Reply