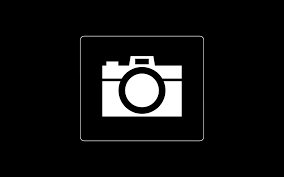
One of the features we use the most in mobile apps is the camera feature. It is the features we use the most, whether you need to take pictures or shoot video. In Xamarin Forms, you need to load a few packages to open the camera. Open the project and right click on the solution and click on Manage Nuget Packages For Solutions. In the page that opens, we are going to upload the package for the camera from the Browse tab. This package is the Xam.Plugin.Media package that James Montemagno has released.
Of course we need to take photos and videos and have a few permissions to keep it. We need to get a few permits for this. If we need to allow the device first, we need to right click on the properties and set the permissions. We need READ_EXTERNAL_STORAGE permission to select the camera permission for the camera, or WRITE_EXTERNAL_STORAGE permission to save the video and photos we have taken to the gallery.
After installing the package I will deal with 4 basic operations.
- Taking pictures from a camera
- Select photos from the gallery
- Video capture from camera
- Choose a video from the gallery
I will open a sample page and design it according to my own wishes. This page will have 4 buttons. These buttons are assigned to perform 4 basic operations. The image control that will be found at the bottom of the page has been put in order to show the photograph we have chosen from the gallery.
<StackLayout> <Button x:Name="btnTakePhoto" Text="Fotoğraf Çek" Clicked="btnTakePhoto_Clicked"/> <Button x:Name="btnPickPhoto" Text="Fotoğraf Seç" Clicked="btnPickPhoto_Clicked"/> <Button x:Name="btnTakeVideo" Text="Video Çek" Clicked="btnTakeVideo_Clicked"/> <Button x:Name="btnPickVideo" Text="Video Seç" Clicked="btnPickVideo_Clicked"/> <Image x:Name="image"/> </StackLayout> </ContentPage.Content>
We will write what happens when you add 4 buttons and click.
To take pictures from the camera, we first need to check whether the camera’s condition is available. It may be used by another application. Afterwards, we check that the camera can not take pictures. If it is not available, the camera will give an error message. If it is already available, we save it to a file. We do this by means of the package we have installed. Afterwards, if a file file is empty, we allow the function to exit. If an image has arrived in the file, we get it with image stream control. We also write where the photo is recorded and what the name will be and finish the process.
private async void btnTakePhoto_Clicked(object sender, EventArgs e) { if (!CrossMedia.Current.IsTakePhotoSupported || !CrossMedia.Current.IsCameraAvailable) { DisplayAlert("HATA", "Maalesef bir sorunla karşılaştık", "OK"); return; } var file = await CrossMedia.Current.TakePhotoAsync(new Plugin.Media.Abstractions.StoreCameraMediaOptions { Directory = "Sample", Name = "photo.jpg", }); if (file == null) { return; } image.Source = ImageSource.FromStream(() => { var stream = file.GetStream(); file.Dispose(); return stream; }); }
In order to take pictures from the gallery, we check the availability of the camera again. If it is not available we will give a camera error message and exit the function. If the camera is available, we assign the file variable to the gallery. If the file is not empty, ie if a photo is selected, we show it under image control.
private async void btnPickPhoto_Clicked(object sender, EventArgs e) { if (!CrossMedia.Current.IsPickPhotoSupported) { DisplayAlert("HATA", "Maalesef telefonunuz fotoğraf seçmeyi desteklemiyor", "OK"); } // Seçtiğimiz fotoğrafı file değişkenine veriyorum var file = await CrossMedia.Current.PickPhotoAsync(); if (file==null) { return; } image.Source = ImageSource.FromStream(() => { var stream = file.GetStream(); file.Dispose(); return stream; }); }
In case of video recording, we check the availability of the camera as usual. If there is an error, we inform the user with the error message. Then we shoot the video variable from the camera. Here we assign this video quality and name, and we decide which format it will be in. If the file is not empty, so if a video is assigned we can show it in a video player. Then we dispatch the file file.
private async Task btnTakeVideo_ClickedAsync(object sender, EventArgs e) { if (!CrossMedia.Current.IsTakePhotoSupported || !CrossMedia.Current.IsCameraAvailable) { DisplayAlert("Hata", "Maalesef kamera şu an da müsait değil", "OK"); return; } var file = await CrossMedia.Current.TakeVideoAsync(new Plugin.Media.Abstractions.StoreVideoOptions { Quality = Plugin.Media.Abstractions.VideoQuality.High, Directory = "SampleVideo", Name = "video.mp4" }); if (file == null) { return; } file.Dispose(); }
In the video selection process, we check whether the gallery is still suitable for selecting videos from the gallery in a classicized way. If not, we display an error message on the screen. If it is available, we are watching the video variable we selected from the gallery. Then we check the file variable and if it is empty, if it is full, we try to show it in a video player if a video is selected.
private async void btnPickVideo_Clicked(object sender, EventArgs e) { if (!CrossMedia.Current.IsPickVideoSupported) { DisplayAlert("Hata", "Video Seçimi Yapamazsınız!", "Tamam"); return; } var file = await CrossMedia.Current.PickVideoAsync(); if (file == null) { return; } file.Dispose(); }
It’s that easy to get images from the camera and choose files from the gallery on Xamarin Forms. There is also a subject that needs to be taken care of, as all functions are async. Once you’ve paid attention to it, you can do things easily.
If you have a problem, you can reach us by email or comment.
Comments (5)
HRN_YLCsays:
Monday April 23rd, 2018 at 02:42 PMÖnem Derecesi Kod Açıklama Proje Dosya Çizgi Gizleme Durumu
Hata invalid resource directory name: obj\Debug\res file_paths.xml “res file_paths.xml”. Kamera.Android C:\Users\Kamera.Android\aapt.exe
omersezersays:
Monday April 23rd, 2018 at 04:04 PMResources klasorunun altinda xml adli yeni bir klasor olusturun. Xml klasorunun icine file_paths.xml dosyasini yapistirin. Yine hata alirsaniz ornek bir projeyi githuba atarim.
HRN_YLCsays:
Friday May 4th, 2018 at 08:18 AMJava.Lang.NullPointerException: Attempt to invoke virtual method ‘void android.support.v7.widget.ContentFrameLayout.setId(int)’ on a null object reference
projeyi githuba atarsanız çok yardımcı olursunuz teşekkürler
omersezersays:
Sunday May 6th, 2018 at 04:47 PMBuyurun projeye buradan ulaşabilirsiniz: https://github.com/omersezer/Xamarin-Forms-Kamera
HRN_YLCsays:
Monday May 14th, 2018 at 04:24 PMÇOK TEŞEKKÜR EDERİM