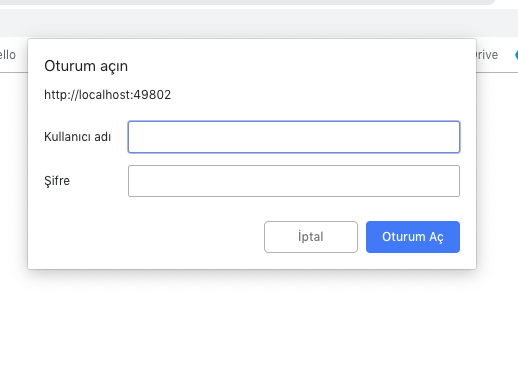
Hello friends. In this article, we will talk about how to authenticate in a web application developed with Asp.Net Core. Authentication can save lives in some cases. It is very important for security that only people who know the username and password can access it.
You need to add a service that comes in the Startup class of your project. We do this simply with the AddAuthentication function. After that, we need to make some adjustments. You can give a name. After naming, you can ask for a simple verification. All you need here is username and password. It’s that simple actually. If you want, you can also give an error message if there is an incorrect entry.
services .AddAuthentication(BasicAuthenticationDefaults.AuthenticationScheme) .AddBasicAuthentication( options => { options.Realm = "My Application"; options.Events = new BasicAuthenticationEvents { OnValidatePrincipal = context => { if ((context.UserName == "username") && (context.Password == "password")) { var claims = new List<Claim> { new Claim(ClaimTypes.Name, context.UserName, context.Options.ClaimsIssuer) }; var principal = new ClaimsPrincipal(new ClaimsIdentity(claims, BasicAuthenticationDefaults.AuthenticationScheme)); context.Principal = principal; } else { // optional with following default. // context.AuthenticationFailMessage = "Authentication failed."; } return Task.CompletedTask; } }; });
Of course, it’s not just limited to these. Maybe you want authentication on some pages and not on other pages. For this, you need to give a feature at the beginning of that page according to which pages you want to use it. This is stated in the code snippet below.
[Authorize] public class HomeController : Controller {
Just write Authorize at the beginning. After that, you will see such a screen on the sites you have Authorized.
If you have questions, you can reach us by sending an e-mail or comment. Enjoy your work.
Leave a Reply