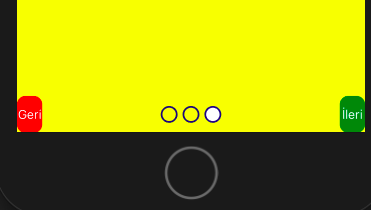
Hello friends. In this article, when the application is opened, we will make a screen that introduces the application. When you try to search on the Internet, you can see it as a Welcome Slider. Someone installing your app; Normally there is a Nuget package for everything but there wasn’t for this when you first open the app. It’s actually a bit complex to make. We will solve this problem with Absotute Layout, Carousel View and a few Grids inside. I will create it from 3 pages to be an example.
First I throw an Absolute Layout. This covers the entire screen. Then I throw a Carousel View into this layout. I can show different screens by turning it sideways with Carousel View. Since I give the Carousel View to the whole screen, I provide a page transition view. If you want, you can fill in the Data Template according to you. There are 5 buttons at the bottom of the page. 2 of these buttons are for back and forth. The other 3 buttons show which page we are on.
<AbsoluteLayout VerticalOptions="FillAndExpand"> <carousel:CarouselView x:Name="carouselView" ItemsSource="{Binding .}" IsNativeStateConsistent="true" PositionSelected="Handle_PositionSelected" IsInNativeLayout="true" AbsoluteLayout.LayoutBounds="0,0,1,1" AbsoluteLayout.LayoutFlags="HeightProportional, WidthProportional"> <carousel:CarouselView.ItemTemplate> <DataTemplate> <Grid BackgroundColor="{Binding BackgroundColor}"> <Grid.RowDefinitions> <RowDefinition Height="*"/> <RowDefinition Height="*"/> <RowDefinition Height="*"/> </Grid.RowDefinitions> <Label Text="{Binding Name}" HorizontalOptions="Center" VerticalOptions="Center" /> </Grid> </DataTemplate> </carousel:CarouselView.ItemTemplate> </carousel:CarouselView> <Grid AbsoluteLayout.LayoutBounds="0,1,1,1" AbsoluteLayout.LayoutFlags="HeightProportional, WidthProportional" VerticalOptions="EndAndExpand" > <Grid.ColumnDefinitions> <ColumnDefinition Width="Auto"/> <ColumnDefinition Width="*"/> <ColumnDefinition Width="Auto"/> </Grid.ColumnDefinitions> <Button Text="Geri" TextColor="White" BorderRadius="10" Grid.Column="0" x:Name="buttonGeri" Clicked="buttonGeri_Clicked" BackgroundColor="Red" /> <Grid Grid.Column="1" HorizontalOptions="Center"> <Grid.ColumnDefinitions> <ColumnDefinition Width="*"/> <ColumnDefinition Width="*"/> <ColumnDefinition Width="*"/> </Grid.ColumnDefinitions> <StackLayout Grid.Column="0" HorizontalOptions="Center" VerticalOptions="Center"> <Button BackgroundColor="Transparent" HeightRequest="20" WidthRequest="20" x:Name="button1" CornerRadius="10" BorderWidth="2" BorderColor="Navy"/> </StackLayout> <StackLayout Grid.Column="1" HorizontalOptions="Center" VerticalOptions="Center"> <Button Grid.Column="1" BackgroundColor="Transparent" HeightRequest="20" WidthRequest="20" x:Name="button2" CornerRadius="10" BorderWidth="2" BorderColor="Navy"/> </StackLayout> <StackLayout Grid.Column="2" VerticalOptions="Center" HorizontalOptions="Center"> <Button BackgroundColor="Transparent" HeightRequest="20" x:Name="button3" WidthRequest="20" CornerRadius="10" BorderWidth="2" BorderColor="Navy"/> </StackLayout> </Grid> <Button Text="İleri" TextColor="White" x:Name="buttonIleri" Clicked="buttonIleri_Clicked" BorderRadius="10" Grid.Column="2" BackgroundColor="Green" /> </Grid> </AbsoluteLayout>
Once you’ve done the design part, it’s necessary to give it a function. For this, we need to switch to the cs side. Of course, first I need to create a model. I’m creating this model to show what will happen on the screen. For simplicity I will change the backgrounds of the screens and just put different text on the screen. I create a list and add 3 yields to this list. Then I bind this list into my Carousel View.
I need to add functionality to the forward and back buttons. I am checking the position of Carousel View. If it is not more than the one in the list, I increase the position of the Carousel View by one and change the back panes of the round buttons that I put at the bottom of the screen to inform the user which page they are on. The forward and back buttons are very similar.
Then, when the Carousel View is moved sideways, we can get the design we want after changing the backgrounds of the round buttons.
public partial class MainPage : ContentPage { List<Model> liste; public MainPage() { InitializeComponent(); liste = new List<Model> { new Model { Name = "Omer", BackgroundColor = "Black" }, new Model { Name = "Ebu", BackgroundColor = "Green" }, new Model { Name = "Barış", BackgroundColor = "Yellow" } }; carouselView.BindingContext = liste; ChangeBackground(carouselView); } void buttonIleri_Clicked(object sender, System.EventArgs e) { if (carouselView.Position +1 !=liste.Count) { carouselView.Position = carouselView.Position + 1; ChangeBackground(carouselView); } } void buttonGeri_Clicked(object sender, System.EventArgs e) { if (carouselView.Position != 0) { carouselView.Position = carouselView.Position - 1; ChangeBackground(carouselView); } } void ChangeBackground(CarouselView carouselView) { switch (carouselView.Position) { case 0: { button1.BackgroundColor = Color.White; button2.BackgroundColor = Color.Transparent; button3.BackgroundColor = Color.Transparent; break; } case 1: { button1.BackgroundColor = Color.Transparent; button2.BackgroundColor = Color.White; button3.BackgroundColor = Color.Transparent; break; } case 2: { button1.BackgroundColor = Color.Transparent; button2.BackgroundColor = Color.Transparent; button3.BackgroundColor = Color.White; break; } default: { button1.BackgroundColor = Color.White; button2.BackgroundColor = Color.Transparent; button3.BackgroundColor = Color.Transparent; break; } } } void Handle_PositionSelected(object sender, Xamarin.Forms.SelectedPositionChangedEventArgs e) { ChangeBackground(carouselView); } }
An example of the project is as follows.
If you have questions, you can reach us by sending an e-mail or comment.
Comments (1)
batuhansays:
Saturday December 28th, 2019 at 11:05 AMpeki bunu uygulama sadece ilk kurulduktan sonra mı gösteriyor yoksa her açıldığında mı?